When developers create React app, they aim to deliver a functional product without bugs that meets the expectations of today’s demanding users. To achieve this, thorough testing and debugging of failing tests are necessary before software release.
In the case of a React application, popular libraries like React Testing Library (RTL) are used for this purpose, alongside popular frameworks like Jest, Vitest, and others.
What Is React Testing and Why Is It Crucial?
React Testing is the process of assessing the functionality of your React app, ensuring that the product performs as expected. The uniqueness of React apps lies in their composition of specific elements called components.
The proper visual illustration below is from the 🔗 freecodecamp.org article Learn React Basics in 10 Minutes Also a valuable part of React Documentation: Component Hierarchy
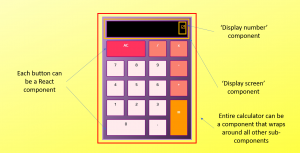
Therefore, the quality control of React applications revolves around testing both internal and external states and the quality of interactions between these components.
Testing your React app enables you to:
- Verify that the digital solution functions properly.
- Make the application less prone to glitches and errors.
- Guarantee a positive user experience.
- Ensure code reliability and product functionality.
👉 Let’s discuss the approaches that are used while testing react components.
Common Approaches to Test React Apps
To maximize the effectiveness of React testing, QA teams employ the following practices:
#1. Test more functionalities, fewer implementation details
Test the complete functionality, not the implementation of its individual details. For example, tests that check the presence of certain attributes work successfully, however, the functionality doesn’t work as the end user would expect.
#2. Emphasis on integration testing
As mentioned earlier, all React applications consist of multiple elements. The challenge is that these components might function correctly on their own but interact incorrectly with each other.
Therefore, integration testing is essential for React digital solutions and other types of tests. This type ensures that all React components work properly regardless of the scenario, including interactions with third-party APIs.
#3. Testing one element at a time
Before writing tests to check specific component elements of your digital solution, create a list of functions that need testing. This approach aids in conducting comprehensive testing, ensuring nothing important is overlooked.
At the same time, this method reduces the size of test suites and speeds up the detection of even minor changes in the code base.
#4. Eliminating unnecessary tests
To justify the importance of this practice, we propose to consider an example test code, with the help of which a tester has to check whether a modal window displays a certain message:
Certainly, achieving high test coverage is a crucial task for QA teams. However, this doesn’t apply to unnecessary tests, which only consume testers’ time and divert their attention from critical scenarios.
How to Test a React App?
To assess the functionality of React apps, several methods of testing. QA engineers can test small code blocks or examine more comprehensive aspects of the program. Below, you’ll find a list of types of React component testing.
- Module Testing. The objective of module tests is to examine small fragments of your code. They allow you to test individual app functions but will never provide a complete picture of the entire product’s performance. These tests are crucial because they enhance the architecture of the digital solution. By conducting them, the IT team can plan the app’s functionality at early development stages, eliminating the need for future code changes.
Interconnections within React modules - Integration Testing. Integration tests enable the assessment of how well React components interact with each other. Despite being costlier and more complex than the previous type of testing, a comprehensive evaluation of a digital product is impossible without it.
→ As part of the big-bang approach, all modules must be integrated together and tested as a single unit. The Big Bang Integration testing practice involves connecting all units at the same time and producing a complete system in the end.
→ Top-down testing. Tests are performed incrementally from the topmost to the lowest level modules using the top-down approach. Testing for each component is done one by one, then the components are integrated to see how it functions as a whole.
→ The bottom-up method tests the lower-level modules first, and then gradually moves to the higher-level modules. It is suitable for testing all units when they are all available.
→ Hybrid integration testing, also known as sandwich testing, combines both bottom-up testing and top-down testing methods, making the process more precise and efficient. A combination of stubs and drivers is used.
- End-to-End (E2E) Testing. In contrast to integration tests, which focus on assessing the interaction of individual system modules, E2E tests are designed to evaluate the functioning of the entire app. They simulate real user scenarios and test the program’s performance not within the development environment but under conditions that closely resemble real user behavior.
Depending on the type of testing required at a specific development stage, QA teams choose specific frameworks and libraries.
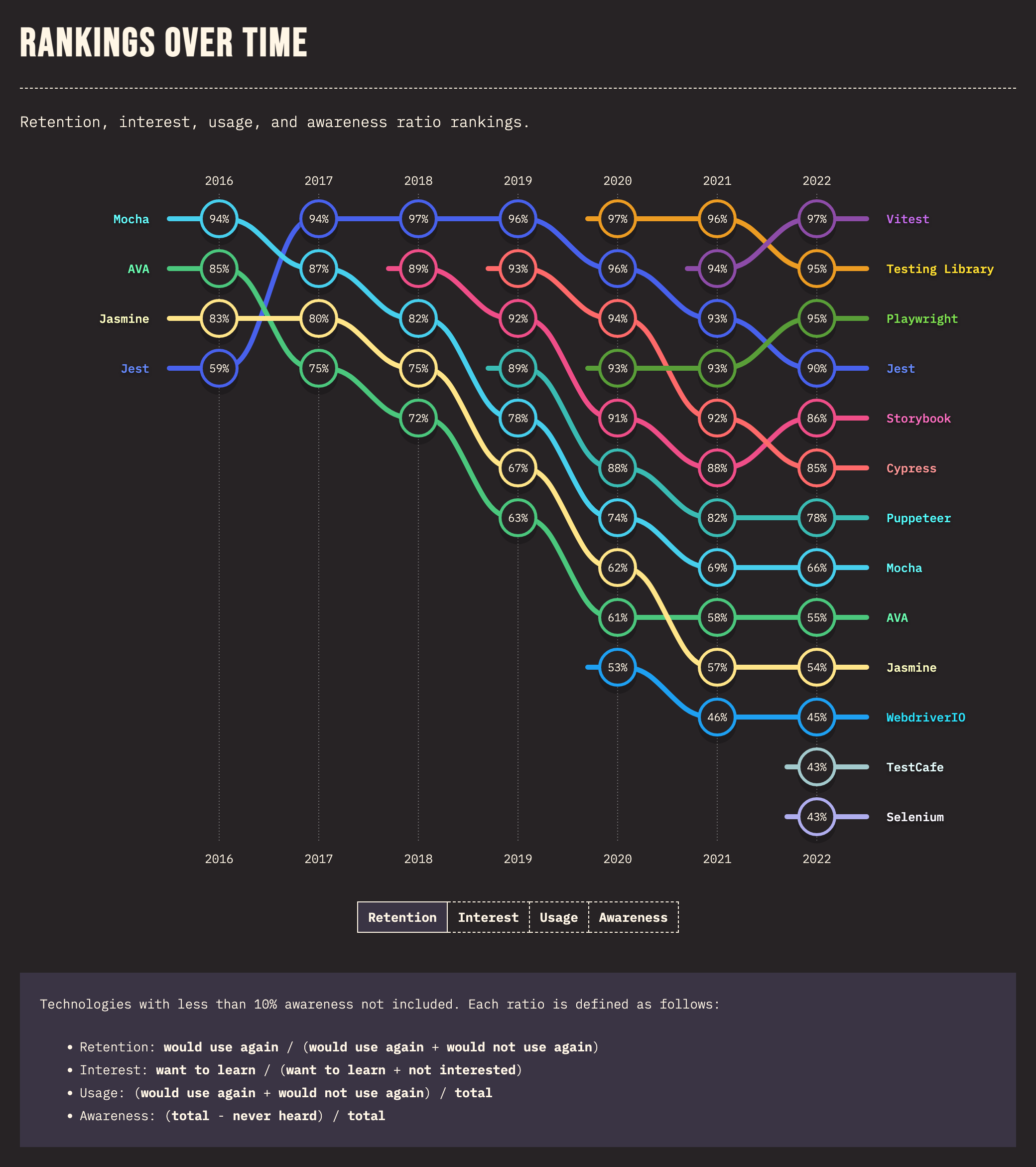
Allow us to consider the most popular ones below ⬇️
Top React Testing Libraries, Frameworks
Testing Framework is a tool that allows testers to create standard test scenarios and perform quality checks on software. They help improve efficiency and yield more accurate results. For module and integration React testing, the following frameworks are widely used:
Jest
Jest is the most popular testing framework for testing React apps, recommended as the RTL by the React community. It is open-source and primarily used for quality checks of web applications.
You can test individual software elements, API components, and user interactions with Jest. Beyond extensive React app testing capabilities, the tool offers other advantages like high performance, compatibility with various applications (React, Angular, VueJS, Node, etc.), and support for snapshots.
To get started with Jest, you’ll need to install NodeJS and package manager first. You can install Jest using your favorite package manager: npm, yarn or pnpm. Find out how in the Official Jest Documentation. Also, our team prepared for you:
A comprehensive tutorial on how to organize an advanced Jest testing framework
Vitest
Vitest is a unit-powered framework designed for module testing and is known for its high-speed execution of tests. It is built upon the Vite build tool, which is well-suited for React projects.
To work with Vitest, you need to install Vite version v3.0.0 or later and Node starting from version v14.18. Then, install the framework using npm, yarn, or pnpm according to the Official Test Framework Guide. You can try Vitest online on StackBlitz.
In addition to frameworks, testing libraries are also used for React app testing. Here are some of the most popular ones:
RTL
React Testing Library is a library for testing React applications, focusing on checking specific component elements from an end-user’s perspective. Its main purpose is to evaluate user behavior rather than delving into the implementation and logic of React components. RTL can be seen as a set of functions used for running tests alongside test frameworks like Jest and Mocha.
Many industry giants utilize RTL to ensure the correctness of user interfaces in their React apps. For example, Airbnb and Facebook’s IT teams use this testing library. Their choice is justified as React Testing Library lets you write more reliable tests because they do not concern the internal workings of the component and allow you to evaluate the performance of the software product in various scenarios, as close as possible to the real conditions of use.
React Testing Library is a testing tool that allows you to create quality user-centered software.
To start React testing with RTL, you must first add the library to your project. If you’re using NPM, enter the following command in the terminal window:
npm install –save-dev @testing-library/react
If you’re using the Yarn package manager, use the following command:
yarn add –dev @testing-library/react
After these steps, you can begin writing tests for your React application. As usual more details in Docs
Also, don’t miss Robin Wieruch’s tutorials for Testing React Apps. It’s great!
Enzyme
Enzyme is a JavaScript testing library primarily used for conducting module tests on React applications. It pairs well with the most popular testing environment for such products – Jest. It’s also compatible with other frameworks like Mocha and Chai.
Compared to RTL discussed earlier, Enzyme’s testing approach is somewhat different. Enzyme code involves props and states, so writing tests happens more from the developer’s point of view. On the other hand, the React Testing Library is closer to real user interaction.
Requirements for working with Enzyme include installing NodeJS and npm. To install the library itself, use the command:
npm i --save-dev enzyme enzyme-adapter-react-16
Finally, you need to configure enzyme like:
import React from 'react';
import sinon from 'sinon';
import { expect } from 'chai';
import { mount } from 'enzyme';
To know more details deep in Documentation
Many testers opt for Enzyme due to its strengths, including:
- Plugin support
- Usage of shallow rendering – a method that allows testing the entire component without delving into deeper levels and without utilizing the DOM
- Support for full DOM rendering – a method crucial for thorough component testing throughout its entire lifecycle.
Useful React Test Utils and test renderer
React Test Utils are utilities that make it easy to test React components in any testing environment chosen by the QA specialist. You may import them using the commands:
import ReactTestUtils from 'react-dom/test-utils';
React Test Renderer is a package that allows you to render a React component without interacting with the browser. It simplifies obtaining instant snapshots in the Jest testing environment. To import React Test Renderer, use the command:
import TestRenderer from 'react-test-renderer';
Some auxiliary tools are also used for React testing:
- Jest Debug plugins VSCode – a tool to debug Jest tests, helping identify reasons for test failures.
- JestLint – a linter for creating better Jest snapshots.
- WebStorm and IntelliJ IDEA – integrated development environments supporting React and offering capabilities for writing and testing applications.
We’ve covered frameworks and libraries for module and integration testing. Let’s now move on to tools well-suited for running E2E tests.
Cypress
Cypress is a framework that enables end-to-end testing of the external interfaces of web apps. You can run and debug tests directly in the browser as fast as the browser can render content. Preparing for testing with Cypress is also time-efficient; writing tests is available without additional testing tools installation and configuration.
To get started with Cypress, meet our article:
The Ultimate Cypress Tutorial: How to Organize your Advanced Testing Framework
Playwright
Playwright is another framework designed for headless browser-based web testing. Playwright is popular among QA teams because it:
- Supports cross-browser and cross-platform testing.
- Lets you run tests in an isolated environment.
- Provides the ability to interact with the software as a real user.
- Enables test script debugging.
To use the framework, install Node.js version 12 or higher and npm, then install Playwright using your favorite package manager: npm, yarn or pnpm. The same you can follow through the Playwright’s resources.
Playwright Testing Tutorial on How to Organize an Advanced (Scaled) (e2e/unit) Testing Framework
CodeceptJS
CodeceptJS is a modern End-to-End testing framework that supports writing tests in BDD format, which speeds up the software quality assurance process. CodeceptJS can be used for web, mobile, and hybrid projects, offering testers a range of other advantages. Among them are interactive debugging, the ability to test multiple browser windows, support for working in CI pipelines, and more.
To work with the framework, you also should install Node.js, NPM, and a web browser. The next actions you might explore with our article or CodeceptJS Docs:
Codeceptjs testing tutorial on how to organize an advanced e2e testing framework
Visual Regression Testing
Next, let’s discuss tools used for Visual Regression Testing. Such tests are aimed at checking what the end user will see after any changes are made to the code. This is accomplished by creating screenshots before and after such changes. The following tools are used to implement this testing approach:
- Meticulous: a tool that automates the detection of bugs in the user interface of your digital solution using AI technology. It takes screen snapshots and detects even the slightest visual differences in the UI.
- Applitools: another AI-based visual testing tool that supports cross-browser testing and provides QA engineers with a convenient UI validation tool called Eyes for writing and maintaining tests. This tool maximizes test coverage and reduces testing time.
- Percy: an automated visual testing tool that integrates easily with testing frameworks or directly with your digital solution. With snapshots, Percy captures the smallest code changes and notifies the team.
Static testing tools
Static testing is another type of testing used to ensure the quality of React apps. These tests are focused on maintaining the cleanliness of your codebase. They identify minor errors in the code, such as typos or missing characters. This type of testing assumes that the code will not be executed during the testing process. Among the popular tools for this type of testing are:
- Prettier: a tool that helps teams not worry about code formatting. It automatically fixes incorrect spaces and line breaks and changes incorrectly used symbols; it keeps the codebase clean and consistent.
- ES Lint: a JavaScript app linter that identifies and automatically fixes code issues. It can be run as part of a CI pipeline, used independently of the development environment, and applied to format code using built-in and custom rules.
- Storybook: a tool that allows you to create and test user interfaces in isolation from the app’s business logic, APIs, and other development aspects. It supports various types of testing, including user interaction checks, visual representations, accessibility checks, and visual and static testing.
Record and Playback testing tools
Record and Playback Testing is a low-code methodology used to test React apps’ quality. Its essence lies in recording user actions and the ability to automatically replay them without the tester’s intervention.
The tools for running such tests provide test automation without writing test scripts.
This approach is widely used for testing mobile apps; many analytics tools support the Record and Playback feature. Some of them include:
- UXCam: session replay, heatmaps, and extensive analytical capabilities allow the QA team to see the complete picture of user behavior and understand how they perceive the digital solution.
- Bird Eats Bug: provides teams with tools for quickly detecting bugs and preventing their recurrence. This includes detailed error reports, the ability to reproduce issues, take screenshots, and record videos of the screen.
- Siesta: a tool designed to run modular JavaScript tests and features a powerful event recorder and player. It supports the most popular frameworks, runs tests in browsers and within Node.js, and allows for simulating real user actions.
- LogRocket: a machine learning-based solution that identifies critical user interaction errors. Among the available tools are session replays, powerful analytics, performance monitoring, and more.
Bottom Line
Well-organized React testing is an essential stage in the software development lifecycle of React Apps. It lets you create high-performance, functional software products without bugs, capable of meeting the needs of even the most demanding users. In total it is:
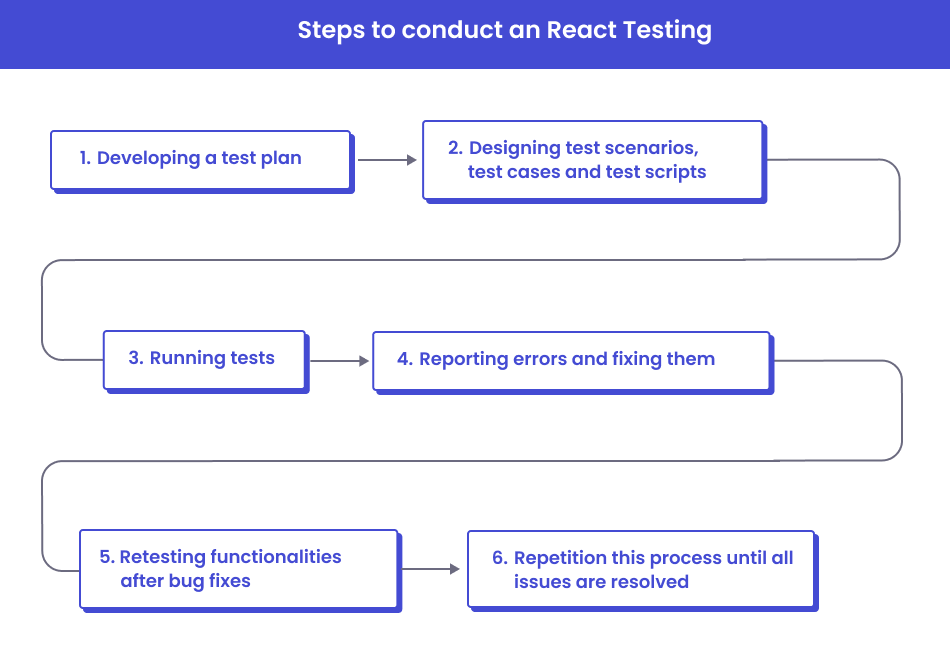
Modern QA teams have access to various frameworks, libraries, and auxiliary utilities, making the testing process highly effective and efficient. When it comes to React testing, testers most commonly rely on frameworks such as Jest, Vitest, Cypress, Playwright, and CodeceptJS, as well as libraries like React Testing Library and Enzyme. But, as we can see numerous tools are also available for static, visual, and Record and Playback Testing.
👉 Let’s know more: What’s The Difference: Integration vs. End-To-End Testing?